Data Structures | |
struct | libinput_device |
A base handle for accessing libinput devices. More... | |
struct | libinput_device_group |
A base handle for accessing libinput device groups. More... | |
struct | libinput_tablet_tool |
An object representing a tool being used by a device with the LIBINPUT_DEVICE_CAP_TABLET_TOOL capability. More... | |
Functions | |
struct libinput_device * | libinput_device_ref (struct libinput_device *device) |
Increase the refcount of the input device. More... | |
struct libinput_device * | libinput_device_unref (struct libinput_device *device) |
Decrease the refcount of the input device. More... | |
void | libinput_device_set_user_data (struct libinput_device *device, void *user_data) |
Set caller-specific data associated with this input device. More... | |
void * | libinput_device_get_user_data (struct libinput_device *device) |
Get the caller-specific data associated with this input device, if any. More... | |
struct libinput * | libinput_device_get_context (struct libinput_device *device) |
Get the libinput context from the device. More... | |
struct libinput_device_group * | libinput_device_get_device_group (struct libinput_device *device) |
Get the device group this device is assigned to. More... | |
const char * | libinput_device_get_sysname (struct libinput_device *device) |
Get the system name of the device. More... | |
const char * | libinput_device_get_name (struct libinput_device *device) |
The descriptive device name as advertised by the kernel and/or the hardware itself. More... | |
unsigned int | libinput_device_get_id_product (struct libinput_device *device) |
Get the product ID for this device. More... | |
unsigned int | libinput_device_get_id_vendor (struct libinput_device *device) |
Get the vendor ID for this device. More... | |
const char * | libinput_device_get_output_name (struct libinput_device *device) |
A device may be mapped to a single output, or all available outputs. More... | |
struct libinput_seat * | libinput_device_get_seat (struct libinput_device *device) |
Get the seat associated with this input device, see Seats for details. More... | |
int | libinput_device_set_seat_logical_name (struct libinput_device *device, const char *name) |
Change the logical seat associated with this device by removing the device and adding it to the new seat. More... | |
struct udev_device * | libinput_device_get_udev_device (struct libinput_device *device) |
Return a udev handle to the device that is this libinput device, if any. More... | |
void | libinput_device_led_update (struct libinput_device *device, enum libinput_led leds) |
Update the LEDs on the device, if any. More... | |
int | libinput_device_has_capability (struct libinput_device *device, enum libinput_device_capability capability) |
Check if the given device has the specified capability. More... | |
int | libinput_device_get_size (struct libinput_device *device, double *width, double *height) |
Get the physical size of a device in mm, where meaningful. More... | |
int | libinput_device_pointer_has_button (struct libinput_device *device, uint32_t code) |
Check if a LIBINPUT_DEVICE_CAP_POINTER device has a button with the given code (see linux/input.h). More... | |
int | libinput_device_keyboard_has_key (struct libinput_device *device, uint32_t code) |
Check if a LIBINPUT_DEVICE_CAP_KEYBOARD device has a key with the given code (see linux/input.h). More... | |
int | libinput_device_switch_has_switch (struct libinput_device *device, enum libinput_switch sw) |
Check if a LIBINPUT_DEVICE_CAP_SWITCH device has a switch of the given type. More... | |
int | libinput_device_tablet_pad_get_num_buttons (struct libinput_device *device) |
Return the number of buttons on a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability. More... | |
int | libinput_device_tablet_pad_get_num_rings (struct libinput_device *device) |
Return the number of rings a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability provides. More... | |
int | libinput_device_tablet_pad_get_num_strips (struct libinput_device *device) |
Return the number of strips a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability provides. More... | |
struct libinput_device_group * | libinput_device_group_ref (struct libinput_device_group *group) |
Increase the refcount of the device group. More... | |
struct libinput_device_group * | libinput_device_group_unref (struct libinput_device_group *group) |
Decrease the refcount of the device group. More... | |
void | libinput_device_group_set_user_data (struct libinput_device_group *group, void *user_data) |
Set caller-specific data associated with this device group. More... | |
void * | libinput_device_group_get_user_data (struct libinput_device_group *group) |
Get the caller-specific data associated with this input device group, if any. More... | |
Detailed Description
Enumeration Type Documentation
◆ libinput_button_state
◆ libinput_device_capability
Capabilities on a device.
A device may have one or more capabilities at a time, capabilities remain static for the lifetime of the device.
◆ libinput_key_state
enum libinput_key_state |
◆ libinput_led
enum libinput_led |
◆ libinput_pointer_axis
Axes on a device with the capability LIBINPUT_DEVICE_CAP_POINTER that are not x or y coordinates.
The two scroll axes LIBINPUT_POINTER_AXIS_SCROLL_VERTICAL and LIBINPUT_POINTER_AXIS_SCROLL_HORIZONTAL are engaged separately, depending on the device. libinput provides some scroll direction locking but it is up to the caller to determine which axis is needed and appropriate in the current interaction
Enumerator | |
---|---|
LIBINPUT_POINTER_AXIS_SCROLL_VERTICAL | |
LIBINPUT_POINTER_AXIS_SCROLL_HORIZONTAL |
◆ libinput_pointer_axis_source
The source for a libinput_pointer_axis event.
See libinput_event_pointer_get_axis_source() for details.
◆ libinput_switch
enum libinput_switch |
The type of a switch.
Enumerator | |
---|---|
LIBINPUT_SWITCH_LID | The laptop lid was closed when the switch state is LIBINPUT_SWITCH_STATE_ON, or was opened when it is LIBINPUT_SWITCH_STATE_OFF. |
LIBINPUT_SWITCH_TABLET_MODE | This switch indicates whether the device is in normal laptop mode or behaves like a tablet-like device where the primary interaction is usually a touch screen. When in tablet mode, the keyboard and touchpad are usually inaccessible. If the switch is in state LIBINPUT_SWITCH_STATE_OFF, the device is in laptop mode. If the switch is in state LIBINPUT_SWITCH_STATE_ON, the device is in tablet mode and the keyboard or touchpad may not be accessible. It is up to the caller to identify which devices are inaccessible in tablet mode. |
◆ libinput_switch_state
The state of a switch.
The default state of a switch is LIBINPUT_SWITCH_STATE_OFF and no event is sent to confirm a switch in the off position. If a switch is logically on during initialization, libinput sends an event of type LIBINPUT_EVENT_SWITCH_TOGGLE with a state LIBINPUT_SWITCH_STATE_ON.
Enumerator | |
---|---|
LIBINPUT_SWITCH_STATE_OFF | |
LIBINPUT_SWITCH_STATE_ON |
◆ libinput_tablet_tool_proximity_state
The state of proximity for a tool on a device.
The device must have the LIBINPUT_DEVICE_CAP_TABLET_TOOL capability.
The proximity of a tool is a binary state signalling whether the tool is within a detectable distance of the tablet device. A tool that is out of proximity cannot generate events.
On some hardware a tool goes out of proximity when it ceases to touch the surface. On other hardware, the tool is still detectable within a short distance (a few cm) off the surface.
Enumerator | |
---|---|
LIBINPUT_TABLET_TOOL_PROXIMITY_STATE_OUT | |
LIBINPUT_TABLET_TOOL_PROXIMITY_STATE_IN |
◆ libinput_tablet_tool_tip_state
The tip contact state for a tool on a device.
The device must have the LIBINPUT_DEVICE_CAP_TABLET_TOOL capability.
The tip contact state of a tool is a binary state signalling whether the tool is touching the surface of the tablet device.
Enumerator | |
---|---|
LIBINPUT_TABLET_TOOL_TIP_UP | |
LIBINPUT_TABLET_TOOL_TIP_DOWN |
◆ libinput_tablet_tool_type
Available tool types for a device with the LIBINPUT_DEVICE_CAP_TABLET_TOOL capability.
The tool type defines the default usage of the tool as advertised by the manufacturer. Multiple different physical tools may share the same tool type, e.g. a Wacom Classic Pen, Wacom Pro Pen and a Wacom Grip Pen are all of type LIBINPUT_TABLET_TOOL_TYPE_PEN. Use libinput_tablet_tool_get_tool_id() to get a specific model where applicable.
Note that on some device, the eraser tool is on the tail end of a pen device. On other devices, e.g. MS Surface 3, the eraser is the pen tip while a button is held down.
- Note
- The libinput_tablet_tool_type can only describe the default physical type of the device. For devices with adjustable physical properties the tool type remains the same, i.e. putting a Wacom stroke nib into a classic pen leaves the tool type as LIBINPUT_TABLET_TOOL_TYPE_PEN.
Function Documentation
◆ libinput_device_get_context()
struct libinput* libinput_device_get_context | ( | struct libinput_device * | device | ) |
Get the libinput context from the device.
- Parameters
-
device A previously obtained device
- Returns
- The libinput context for this device.
◆ libinput_device_get_device_group()
struct libinput_device_group* libinput_device_get_device_group | ( | struct libinput_device * | device | ) |
Get the device group this device is assigned to.
Some physical devices like graphics tablets are represented by multiple kernel devices and thus by multiple struct libinput_device.
libinput assigns these devices to the same libinput_device_group allowing the caller to identify such devices and adjust configuration settings accordingly. For example, setting a tablet to left-handed often means turning it upside down. A touch device on the same tablet would need to be turned upside down too to work correctly.
All devices are part of a device group though for most devices the group will be a singleton. A device is assigned to a device group on LIBINPUT_EVENT_DEVICE_ADDED and removed from that group on LIBINPUT_EVENT_DEVICE_REMOVED. It is up to the caller to track how many devices are in each device group.
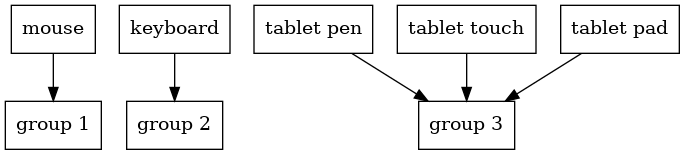
Device groups do not get re-used once the last device in the group was removed, i.e. unplugging and re-plugging a physical device with grouped devices will return a different device group after every unplug.
The returned device group is not refcounted and may become invalid after the next call to libinput. Use libinput_device_group_ref() and libinput_device_group_unref() to continue using the handle outside of the immediate scope.
Device groups are assigned based on the LIBINPUT_DEVICE_GROUP udev property, see Static device configuration via udev.
- Returns
- The device group this device belongs to
◆ libinput_device_get_id_product()
unsigned int libinput_device_get_id_product | ( | struct libinput_device * | device | ) |
Get the product ID for this device.
- Parameters
-
device A previously obtained device
- Returns
- The product ID of this device
◆ libinput_device_get_id_vendor()
unsigned int libinput_device_get_id_vendor | ( | struct libinput_device * | device | ) |
Get the vendor ID for this device.
- Parameters
-
device A previously obtained device
- Returns
- The vendor ID of this device
◆ libinput_device_get_name()
const char* libinput_device_get_name | ( | struct libinput_device * | device | ) |
The descriptive device name as advertised by the kernel and/or the hardware itself.
To get the sysname for this device, use libinput_device_get_sysname().
The lifetime of the returned string is tied to the struct libinput_device. The string may be the empty string but is never NULL.
- Parameters
-
device A previously obtained device
- Returns
- The device name
◆ libinput_device_get_output_name()
const char* libinput_device_get_output_name | ( | struct libinput_device * | device | ) |
A device may be mapped to a single output, or all available outputs.
If a device is mapped to a single output only, a relative device may not move beyond the boundaries of this output. An absolute device has its input coordinates mapped to the extents of this output.
- Note
- Use of this function is discouraged. Its return value is not precisely defined and may not be understood by the caller or may be insufficient to map the device. Instead, the system configuration could set a udev property the caller understands and interprets correctly. The caller could then obtain device with libinput_device_get_udev_device() and query it for this property. For more complex cases, the caller must implement monitor-to-device association heuristics.
- Returns
- The name of the output this device is mapped to, or NULL if no output is set
◆ libinput_device_get_seat()
struct libinput_seat* libinput_device_get_seat | ( | struct libinput_device * | device | ) |
Get the seat associated with this input device, see Seats for details.
A seat can be uniquely identified by the physical and logical seat name. There will ever be only one seat instance with a given physical and logical seat name pair at any given time, but if no external reference is kept, it may be destroyed if no device belonging to it is left.
The returned seat is not refcounted and may become invalid after the next call to libinput. Use libinput_seat_ref() and libinput_seat_unref() to continue using the handle outside of the immediate scope.
- Parameters
-
device A previously obtained device
- Returns
- The seat this input device belongs to
◆ libinput_device_get_size()
int libinput_device_get_size | ( | struct libinput_device * | device, |
double * | width, | ||
double * | height | ||
) |
Get the physical size of a device in mm, where meaningful.
This function only succeeds on devices with the required data, i.e. tablets, touchpads and touchscreens.
If this function returns nonzero, width and height are unmodified.
- Parameters
-
device The device width Set to the width of the device height Set to the height of the device
- Returns
- 0 on success, or nonzero otherwise
◆ libinput_device_get_sysname()
const char* libinput_device_get_sysname | ( | struct libinput_device * | device | ) |
Get the system name of the device.
To get the descriptive device name, use libinput_device_get_name().
- Parameters
-
device A previously obtained device
- Returns
- System name of the device
◆ libinput_device_get_udev_device()
struct udev_device* libinput_device_get_udev_device | ( | struct libinput_device * | device | ) |
Return a udev handle to the device that is this libinput device, if any.
The returned handle has a refcount of at least 1, the caller must call udev_device_unref() once to release the associated resources. See the libudev documentation for details.
Some devices may not have a udev device, or the udev device may be unobtainable. This function returns NULL if no udev device was available.
Calling this function multiple times for the same device may not return the same udev handle each time.
- Parameters
-
device A previously obtained device
- Returns
- A udev handle to the device with a refcount of >= 1 or NULL.
- Return values
-
NULL This device is not represented by a udev device
◆ libinput_device_get_user_data()
void* libinput_device_get_user_data | ( | struct libinput_device * | device | ) |
Get the caller-specific data associated with this input device, if any.
- Parameters
-
device A previously obtained device
- Returns
- Caller-specific data pointer or NULL if none was set
- See also
- libinput_device_set_user_data
◆ libinput_device_group_get_user_data()
void* libinput_device_group_get_user_data | ( | struct libinput_device_group * | group | ) |
Get the caller-specific data associated with this input device group, if any.
- Parameters
-
group A previously obtained group
- Returns
- Caller-specific data pointer or NULL if none was set
◆ libinput_device_group_ref()
struct libinput_device_group* libinput_device_group_ref | ( | struct libinput_device_group * | group | ) |
Increase the refcount of the device group.
A device group will be freed whenever the refcount reaches 0. This may happen during libinput_dispatch() if all devices of this group were removed from the system. A caller must ensure to reference the device group correctly to avoid dangling pointers.
- Parameters
-
group A previously obtained device group
- Returns
- The passed device group
◆ libinput_device_group_set_user_data()
void libinput_device_group_set_user_data | ( | struct libinput_device_group * | group, |
void * | user_data | ||
) |
Set caller-specific data associated with this device group.
libinput does not manage, look at, or modify this data. The caller must ensure the data is valid.
- Parameters
-
group A previously obtained device group user_data Caller-specific data pointer
◆ libinput_device_group_unref()
struct libinput_device_group* libinput_device_group_unref | ( | struct libinput_device_group * | group | ) |
Decrease the refcount of the device group.
A device group will be freed whenever the refcount reaches 0. This may happen during libinput_dispatch() if all devices of this group were removed from the system. A caller must ensure to reference the device group correctly to avoid dangling pointers.
- Parameters
-
group A previously obtained device group
- Returns
- NULL if the device group was destroyed, otherwise the passed device group
◆ libinput_device_has_capability()
int libinput_device_has_capability | ( | struct libinput_device * | device, |
enum libinput_device_capability | capability | ||
) |
Check if the given device has the specified capability.
- Returns
- Non-zero if the given device has the capability or zero otherwise
◆ libinput_device_keyboard_has_key()
int libinput_device_keyboard_has_key | ( | struct libinput_device * | device, |
uint32_t | code | ||
) |
Check if a LIBINPUT_DEVICE_CAP_KEYBOARD device has a key with the given code (see linux/input.h).
- Parameters
-
device A current input device code Key code to check for, e.g. KEY_ESC
- Returns
- 1 if the device supports this key code, 0 if it does not, -1 on error.
◆ libinput_device_led_update()
void libinput_device_led_update | ( | struct libinput_device * | device, |
enum libinput_led | leds | ||
) |
Update the LEDs on the device, if any.
If the device does not have LEDs, or does not have one or more of the LEDs given in the mask, this function does nothing.
- Parameters
-
device A previously obtained device leds A mask of the LEDs to set, or unset.
◆ libinput_device_pointer_has_button()
int libinput_device_pointer_has_button | ( | struct libinput_device * | device, |
uint32_t | code | ||
) |
Check if a LIBINPUT_DEVICE_CAP_POINTER device has a button with the given code (see linux/input.h).
- Parameters
-
device A current input device code Button code to check for, e.g. BTN_LEFT
- Returns
- 1 if the device supports this button code, 0 if it does not, -1 on error.
◆ libinput_device_ref()
struct libinput_device* libinput_device_ref | ( | struct libinput_device * | device | ) |
Increase the refcount of the input device.
An input device will be freed whenever the refcount reaches 0. This may happen during libinput_dispatch() if the device was removed from the system. A caller must ensure to reference the device correctly to avoid dangling pointers.
- Parameters
-
device A previously obtained device
- Returns
- The passed device
◆ libinput_device_set_seat_logical_name()
int libinput_device_set_seat_logical_name | ( | struct libinput_device * | device, |
const char * | name | ||
) |
Change the logical seat associated with this device by removing the device and adding it to the new seat.
This command is identical to physically unplugging the device, then re-plugging it as a member of the new seat. libinput will generate a LIBINPUT_EVENT_DEVICE_REMOVED event and this libinput_device is considered removed from the context; it will not generate further events and will be freed when the refcount reaches zero. A LIBINPUT_EVENT_DEVICE_ADDED event is generated with a new libinput_device handle. It is the caller's responsibility to update references to the new device accordingly.
If the logical seat name already exists in the device's physical seat, the device is added to this seat. Otherwise, a new seat is created.
- Note
- This change applies to this device until removal or libinput_suspend(), whichever happens earlier.
- Parameters
-
device A previously obtained device name The new logical seat name
- Returns
- 0 on success, non-zero on error
◆ libinput_device_set_user_data()
void libinput_device_set_user_data | ( | struct libinput_device * | device, |
void * | user_data | ||
) |
Set caller-specific data associated with this input device.
libinput does not manage, look at, or modify this data. The caller must ensure the data is valid.
- Parameters
-
device A previously obtained device user_data Caller-specific data pointer
- See also
- libinput_device_get_user_data
◆ libinput_device_switch_has_switch()
int libinput_device_switch_has_switch | ( | struct libinput_device * | device, |
enum libinput_switch | sw | ||
) |
Check if a LIBINPUT_DEVICE_CAP_SWITCH device has a switch of the given type.
- Parameters
-
device A current input device sw Switch to check for
- Returns
- 1 if the device supports this switch, 0 if it does not, -1 on error.
◆ libinput_device_tablet_pad_get_num_buttons()
int libinput_device_tablet_pad_get_num_buttons | ( | struct libinput_device * | device | ) |
Return the number of buttons on a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability.
Buttons on a pad device are numbered sequentially, see Tablet pad button numbers for details.
- Parameters
-
device A current input device
- Returns
- The number of buttons supported by the device.
◆ libinput_device_tablet_pad_get_num_rings()
int libinput_device_tablet_pad_get_num_rings | ( | struct libinput_device * | device | ) |
Return the number of rings a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability provides.
- Parameters
-
device A current input device
- Returns
- The number of rings or 0 if the device has no rings.
◆ libinput_device_tablet_pad_get_num_strips()
int libinput_device_tablet_pad_get_num_strips | ( | struct libinput_device * | device | ) |
Return the number of strips a device with the LIBINPUT_DEVICE_CAP_TABLET_PAD capability provides.
- Parameters
-
device A current input device
- Returns
- The number of strips or 0 if the device has no strips.
◆ libinput_device_unref()
struct libinput_device* libinput_device_unref | ( | struct libinput_device * | device | ) |
Decrease the refcount of the input device.
An input device will be freed whenever the refcount reaches 0. This may happen during libinput_dispatch if the device was removed from the system. A caller must ensure to reference the device correctly to avoid dangling pointers.
- Parameters
-
device A previously obtained device
- Returns
- NULL if the device was destroyed, otherwise the passed device